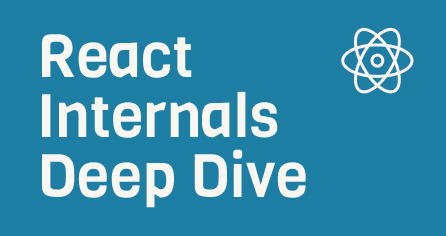
React Internals Deep Dive
A live series of JSer deep diving into React internals, by reading the actual React source code. This series helps you understand how React works internally and write better React code.
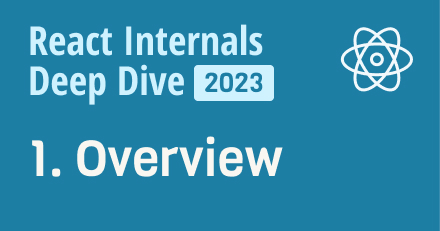
1 - The Overview of React internals
Rough overview of React internals by debugging the simplest Hello World app.
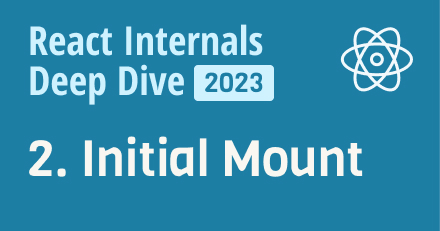
2 - Initial Mount, how does it work?
How React constructs the DOM tree for the initial render.
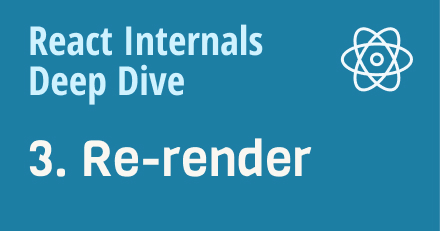
3 - How doest React re-render internally?
How React handles updates and re-renders accordingly.
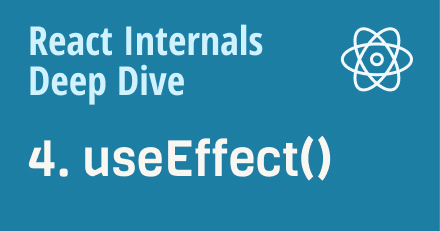
4 - How does useEffect() work internally?
useEffect() is one of the most confusing hooks in React, I'll explain to you how it works internally.
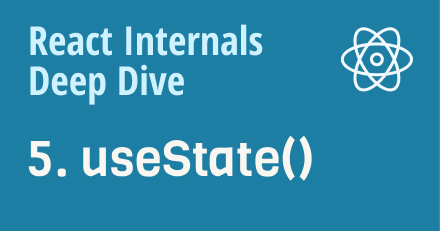
5 - How does useState work internally?
How useState() triggers re-renders in React.
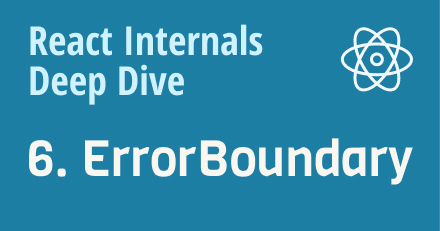
6 - How does ErrorBoundary work internally?
ErrorBoundary is one of the most interesting components I think, this post covers its internals.
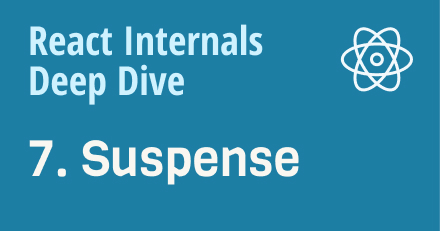
7 - How does Suspense work internally?
Suspense is one of the most important components in React development today, this post covers its internals.
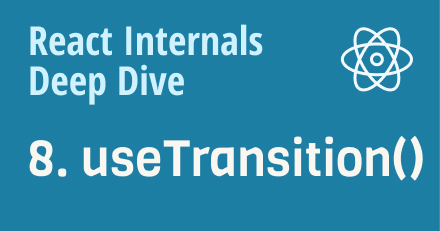
8 - How does useTransition() work internally?
Following Suspense, transition is another advanced topic in React, it is useful to work with React Server Components together.
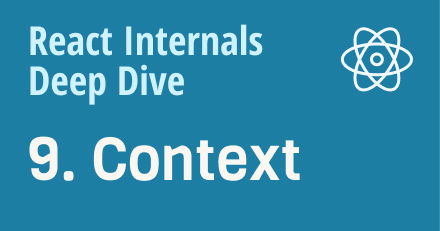
9 - How does Context work internally in React?
Context is a powerful tool in React, I'll explain to you in this post about how it works.
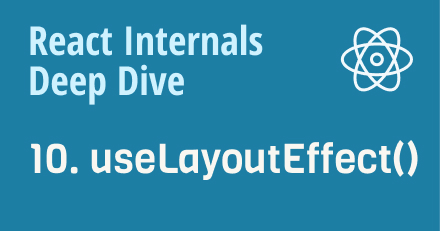
10 - How does useLayoutEffect() work internally?
useLayoutEffect() allows us to run effects before UI painting, have you wondered how it works internally?
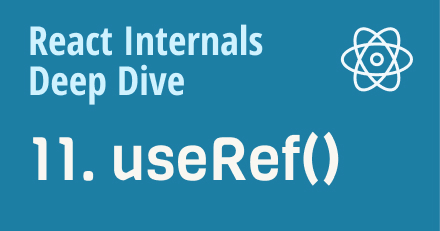
11 - How does useRef() work internally?
useRef() is a powerful yet simple hook in React, I'll explain to you how it works internally in this episode.
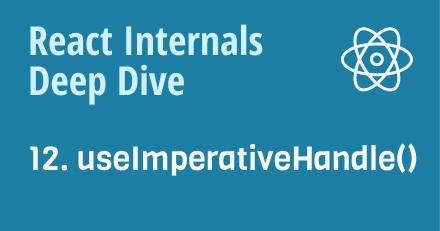
12 - How does useImperativeHandle() work internally?
useImperativeHandle() might not be that commonly used, yet it is interesting to find out how it works internally
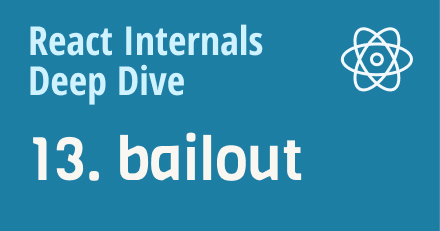
13 - How does React bailout work in reconciliation?
React has "re-render everything" mental model, but it also has this internal optimization of bailout to avoid unecessary render
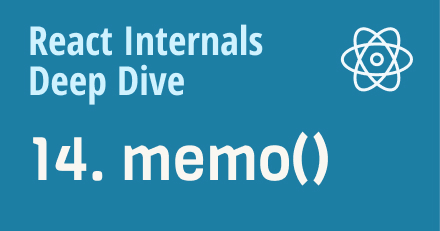
14 - How does React.memo() work internally?
memo() allows us to improve performance by reducing unnecessary re-renders which built-in bailout cannot detect.
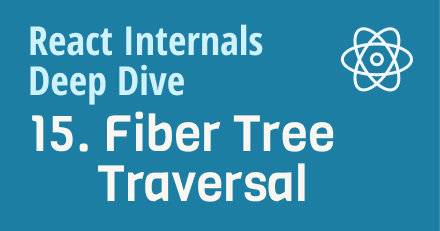
15 - How does React traverse Fiber tree internally?
React holds a fiber tree internally, do you know how it traverse it?
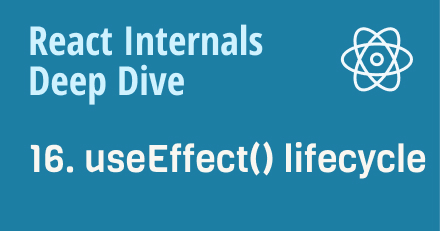
16 - The lifecycle of effect hooks in React
A detailed explanation for the internals of useEffect()
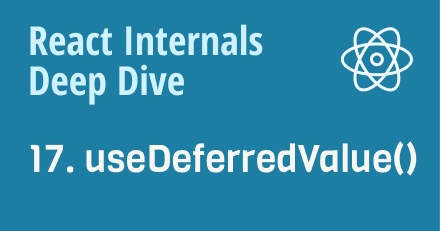
17 - How does useDeferredValue() work internally?
useDeferredValue() is hook to show the power of concurrent mode, let's see how it works internally
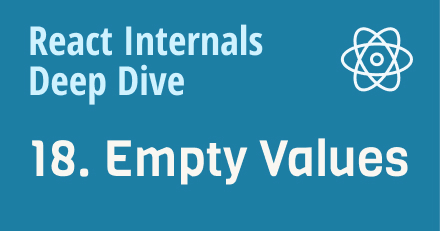
18 - How React handles empty values internally?
Empty values are ignored in react, we'll see how it is achieve internally.
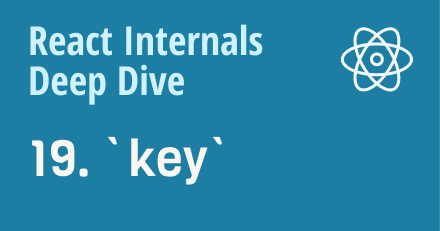
19 - How does 'key' work? List diffing in React
'key' is a powerful hint in React world, do you know how it actually works?
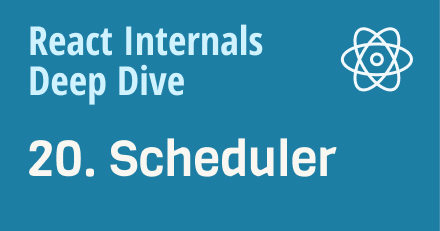
20 - How does React Scheduler work internally?
Scheduler is one of the most important part of React, it is quite useful to understand how it schedules work of different priorities.
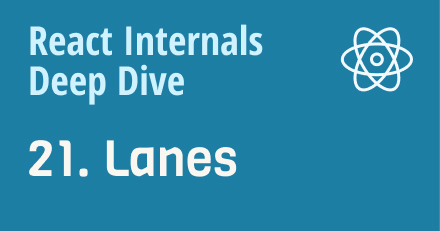
21 - What are Lanes in React source code?
Lanes means priority, let's see what it is and how it enables concurrent mode
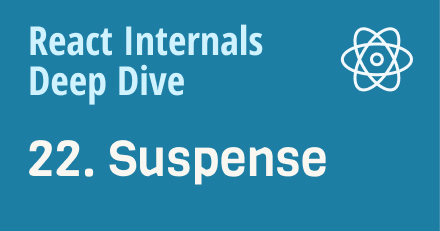
22 - How Suspense works internally in Concurrent Mode 1 - Reconciling flow
Suspense works similar to ErrorBoundary, but way more complex. I'll explain its basic rendering mechanism.
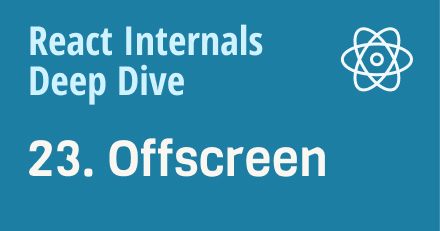
23 - How Suspense works internally in Concurrent Mode 2 - Offscreen component
Offscreen component is used under the hood of Suspense, it is very useful
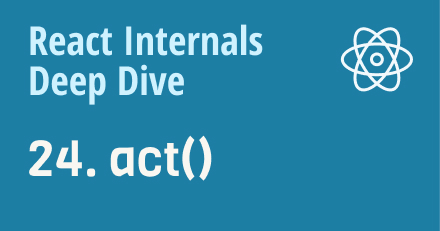
24 - How does act() work internally in React?
act() is useful in React testing, and it helps understand the React scheduler as well.
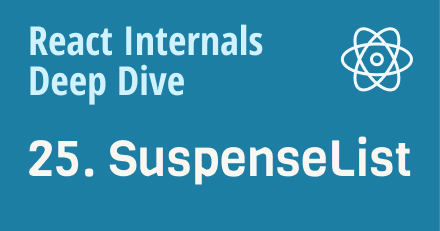
25 - How does SuspenseList work internally in React?
On top of Suspense, SuspenseList is a component to gain better UX
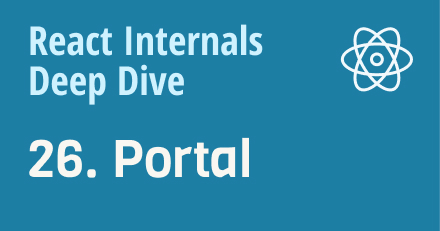
26 - How does React Portal work internally ?
Portal enables rendering React elements to different DOM container, perfect for modals.
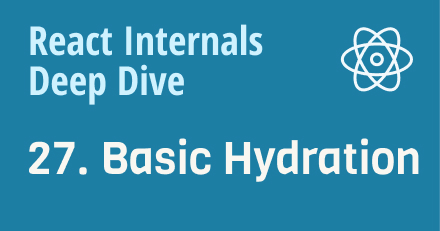
27 - How basic hydration works internally in React ?
Hydration is the process of making server-side rendered DOM interactive, this episode covers the basic case
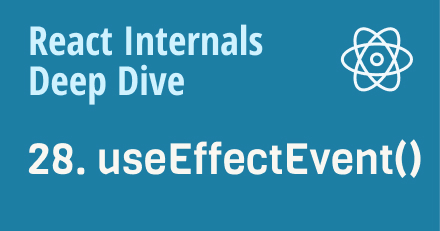
28 - How does useEffectEvent() works internally in React?
useEffectEvent() tries to make it easier to handle event handlers with useEffect().
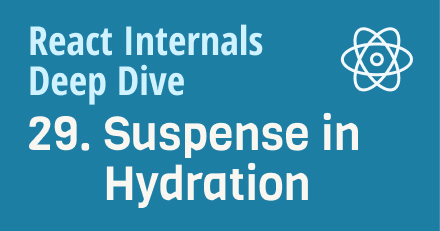
29 - How hydration works with Suspense internally in React?
Server-side rendering is cool, but have you wondered how Suspense is handled?
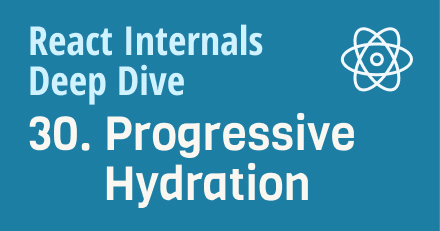
30 - What is Progressive Hydration and how does it work internally in React?
Progressive Hydration is a magic to make Suspense cope with hydration like a charm.
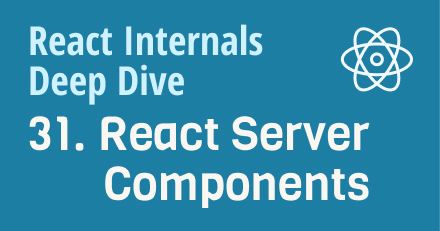
31 - How do React Server Components(RSC) work internally in React?
React Server Components open up new possibilities of React, it is a perfect tool to build fullstack experiences.
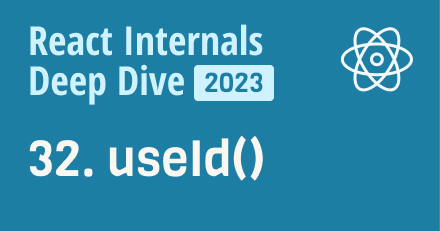
32 - How does useId() work internally in React?
useId() is a simple but quite interesting hook to generate unique ids, let's see how the algorithm works
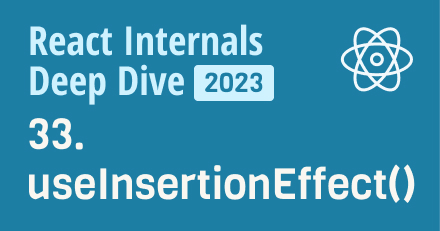
33 - How does useInsertionEffect() work internally in React?
useInsertionEffect() allows us to run some effects before the DOM mutation is done, it is the earliest timing we can get from effect hooks.
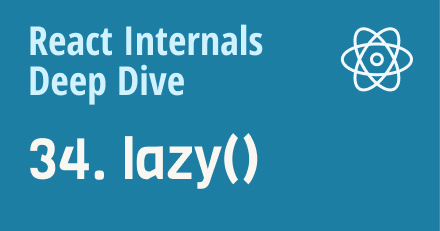
34 - How lazy() works internally in React?
Built-in `lazy()` is an easy way to lazy-load component when rendered, we'll figure out its internals in this episode.
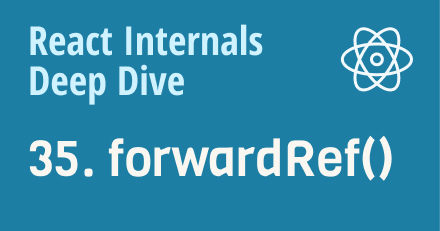
35 - How forwardRef() works internally in React?
forwardRef() in React allows us to create a component in which we can extract ref from props. We are not able to achieve this by ourself because ref is handled specially. Let’s dive into its internals today.
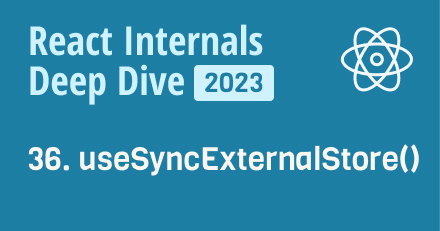
36 - How useSyncExternalStore() works internally in React?
useSyncExternalStore() is a React Hook that lets you subscribe to an external store easily and addresses issues of tearing / undetected data changes
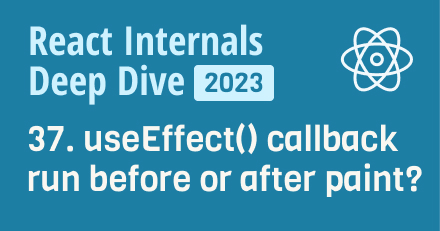
37 - When do useEffect() callbacks get run? Before paint or after paint?
Most of time useEffect() callbacks are run after paint but sometimes before paint.
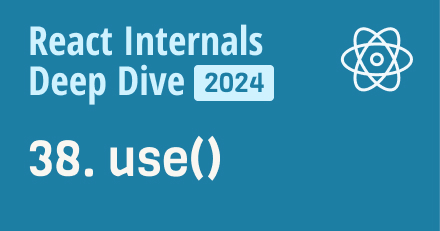
38 - How does use() work internally in React?
use() is an easy way to use Promise or context as resources.
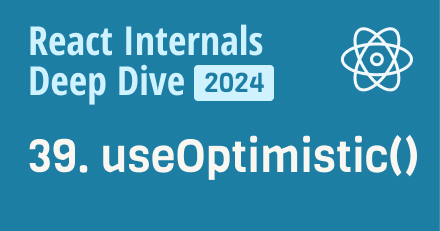
39 - How does useOptimistic() work internally in React?
useOptimistic() helps build optimistic UI to improved perceived performance.